How to Run HTML to PDF with .NET on Azure?
Yes. IronPDF can be used to generate, manipulate, and read PDF documents on Azure. IronPDF has been thoroughly tested on multiple Azure platforms including MVC websites, Azure Functions, and many more.
Azure Functions on Docker
If you are running Azure Functions within a Docker Container please refer to this tutorial instead.
How to Make PDF Generator in Azure Function
- Install C# library to generate PDFs in Azure
- Choose the Azure Basic B1 hosting tier or above
- Uncheck the
Run from package file
option when publish - Follow the recommended configuration instructions
- Use the code example to create PDF generator using Azure
How to Tutorial
Setting up Your Project
Installing IronPDF to Get Started
First step is to install IronPDF using NuGet:
- On Windows based Azure Functions use the
IronPdf
package - https://www.nuget.org/packages/IronPdf/ - On Linux based Azure Functions use the
IronPdf.Linux
package - https://www.nuget.org/packages/IronPdf.Linux/
Install-Package IronPdf
Alternatively, manually install the .dll via direct download link
Select Correct Azure Options
Choosing the correct hosting level Azure Tier
Azure Basic B1 is the minimum hosting level required for our end users' rendering needs. If you are creating a high throughput system, this may need to be upgraded.
Before proceeding
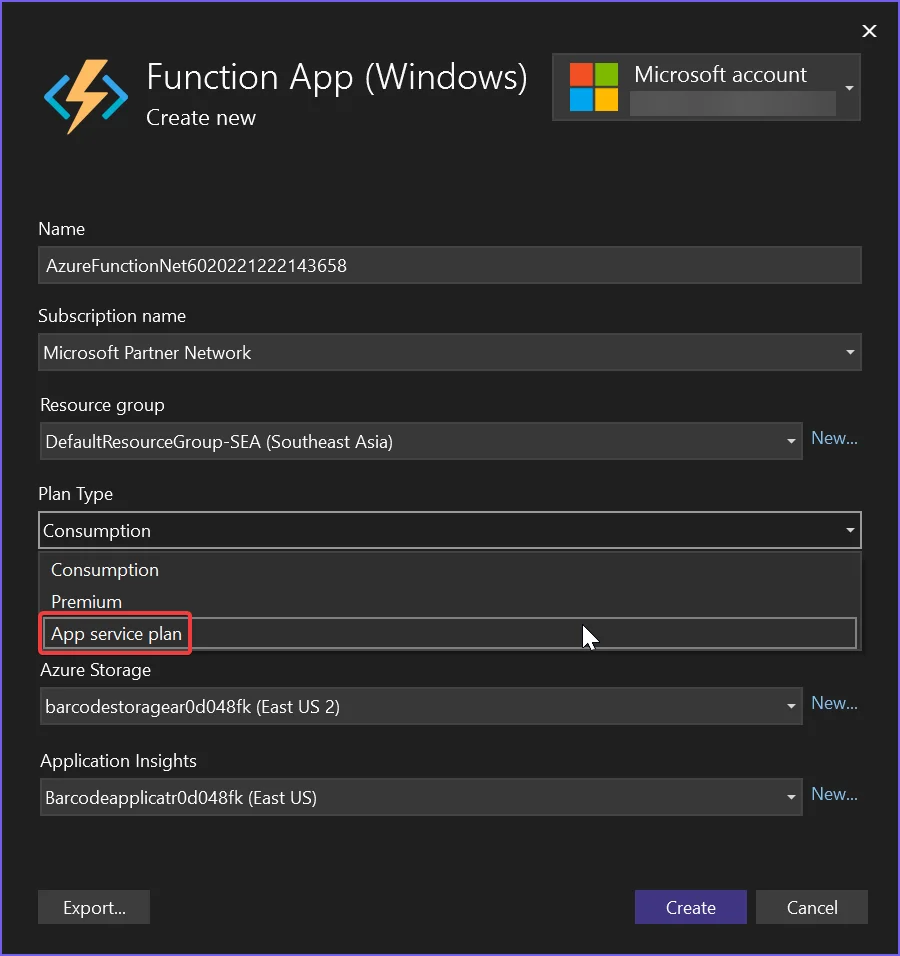
The "Run from package file" Checkbox
When publishing your Azure Functions application make sure Run from package file
is NOT selected.
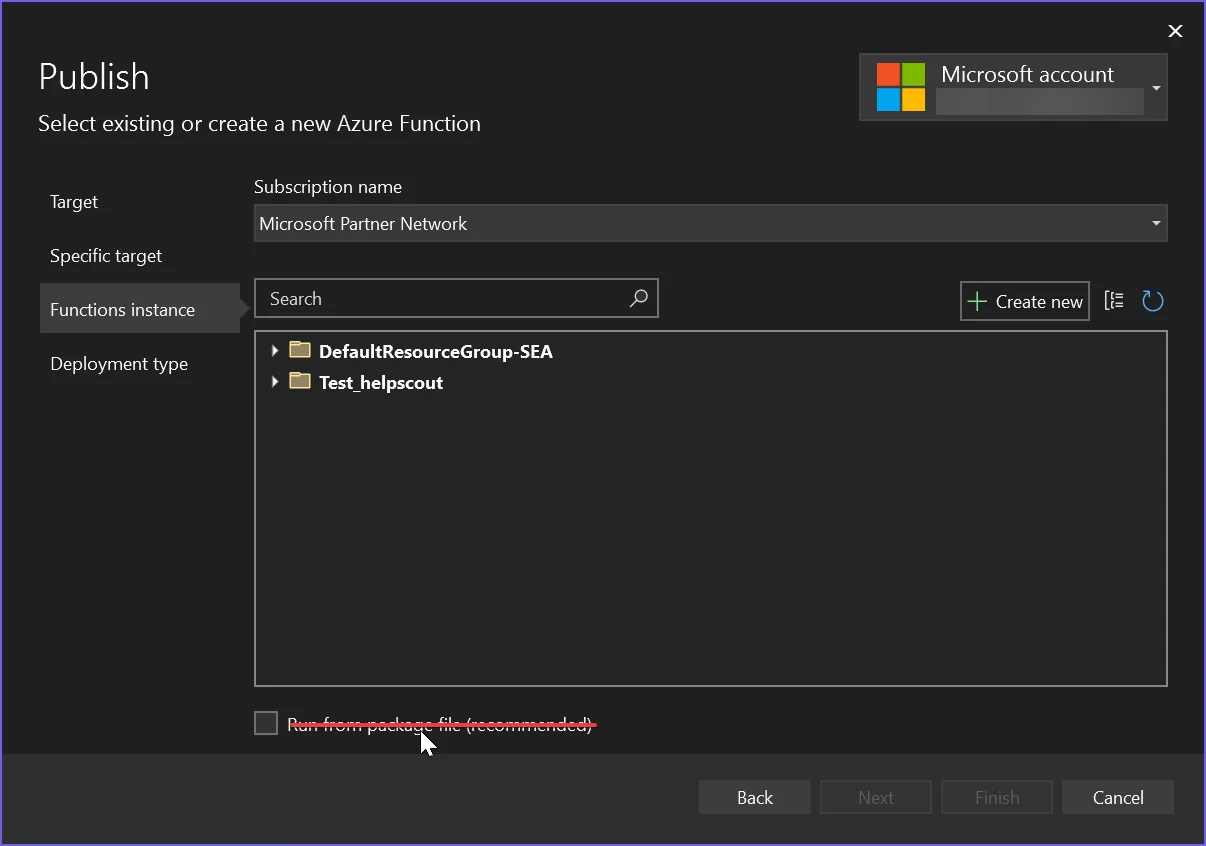
Configuration for .NET 6
Microsoft recently removed imaging libraries from .NET 6+, breaking many legacy APIs. As such, it is necessary to configure your project to still allow these legacy API calls.
- On Linux, set
Installation.LinuxAndDockerDependenciesAutoConfig=true;
to ensurelibgdiplus
is installed on the machine - Add the following to the .csproj file for your .NET 6 project:
<GenerateRuntimeConfigurationFiles>true</GenerateRuntimeConfigurationFiles>
- Create a file in your project called
runtimeconfig.template.json
and populate it with the following:
{
"configProperties": {
"System.Drawing.EnableUnixSupport": true
}
}
{
"configProperties": {
"System.Drawing.EnableUnixSupport": true
}
}
If True Then
"configProperties":
If True Then
"System.Drawing.EnableUnixSupport": True
End If
End If
- Finally, add the following line to the beginning of your program:
System.AppContext.SetSwitch("System.Drawing.EnableUnixSupport", true);
Using Docker on Azure
One way to gain control, SVG font access, and the ability to control performance on Azure is to use IronPDF applications and Functions from within Docker Containers.
We have a comprehensive IronPDF Azure Docker tutorial for Linux and Windows instances and it is recommended reading.
Azure Function Code Example
This example automatically outputs log entries to the built-in Azure logger (see ILogger log
):
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
IronPdf.Installation.LicenseKey = "XXXX"; // you may enter a license key here!
IronPdf.Logging.Logger.EnableDebugging = false;
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
Installation.LinuxAndDockerDependenciesAutoConfig = false;
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
using var doc = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(doc.BinaryData, "application/pdf"){FileDownloadName = "google.pdf"};
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
}
return new OkObjectResult("OK");
}
[FunctionName("PrintPdf")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log, ExecutionContext context)
{
log.LogInformation("Entered PrintPdf API function...");
IronPdf.Installation.LicenseKey = "XXXX"; // you may enter a license key here!
IronPdf.Logging.Logger.EnableDebugging = false;
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom;
IronPdf.Logging.Logger.CustomLogger = log;
Installation.LinuxAndDockerDependenciesAutoConfig = false;
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled;
try
{
log.LogInformation("About to render pdf...");
ChromePdfRenderer renderer = new ChromePdfRenderer();
using var doc = renderer.RenderUrlAsPdf("https://www.google.com/");
log.LogInformation("finished rendering pdf...");
return new FileContentResult(doc.BinaryData, "application/pdf"){FileDownloadName = "google.pdf"};
}
catch (Exception e)
{
log.LogError(e, "Error while rendering pdf", e);
}
return new OkObjectResult("OK");
}
<FunctionName("PrintPdf")>
Public Shared Async Function Run(<HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route := Nothing)> ByVal req As HttpRequest, ByVal log As ILogger, ByVal context As ExecutionContext) As Task(Of IActionResult)
log.LogInformation("Entered PrintPdf API function...")
IronPdf.Installation.LicenseKey = "XXXX" ' you may enter a license key here!
IronPdf.Logging.Logger.EnableDebugging = False
IronPdf.Logging.Logger.LoggingMode = IronPdf.Logging.Logger.LoggingModes.Custom
IronPdf.Logging.Logger.CustomLogger = log
Installation.LinuxAndDockerDependenciesAutoConfig = False
Installation.ChromeGpuMode = IronPdf.Engines.Chrome.ChromeGpuModes.Disabled
Try
log.LogInformation("About to render pdf...")
Dim renderer As New ChromePdfRenderer()
Dim doc = renderer.RenderUrlAsPdf("https://www.google.com/")
log.LogInformation("finished rendering pdf...")
Return New FileContentResult(doc.BinaryData, "application/pdf") With {.FileDownloadName = "google.pdf"}
Catch e As Exception
log.LogError(e, "Error while rendering pdf", e)
End Try
Return New OkObjectResult("OK")
End Function
Known Issues
SVG fonts rendering is not available on shared hosting plans
One limitation we have found is that the Azure hosting platform does not support servers loading SVG fonts, such as Google Fonts, in their cheaper shared web-app tiers. This is because these shared hosting platforms are not allowed to access windows GDI+ graphics objects for security reasons.
We recommend using a Windows or Linux Docker Container or perhaps a VPS on Azure to navigate this issue where the best font rendering is required.
Azure free tier hosting is slow
Azure free and shared tiers, and the consumption plan, are not suitable for PDF rendering. We recommend Azure B1 hosting/Premium plan, which is what we use ourselves. The process of HTML to PDF
is significant 'work' for any computer - similar to opening and rendering a web page on your own machine. A real browser engine is used, hence we need to provision accordingly and expect similar render times to a desktop machine of similar power.
Creating an Engineering Support Request Ticket
In order to create an Engineering Support Request Ticket refer to this guide: https://ironpdf.com/troubleshooting/engineering-request-pdf/