How to Print PDF Files
Sending a PDF to a printer from .NET C# code automates the printing process, allowing you to integrate printing functionality into your applications, reducing manual efforts, and ensuring consistency in PDF files production. It provides precise control over the printing process.
IronPDF offers the option to quickly print programmatically to a physical printer in one method call, allowing you to print multiple PDF files. The printer resolution can also be specified with configurable horizontal and vertical DPI. Use the method that accepts both Microsoft PrinterSettings and PrintController for further control over the PDF printing process.
How to Print PDF File in C
- Download the C# library to print PDF to a physical printer
- Prepare the input PDF file for printing
- Use the
Print
method to send the PDF files to the default printer - Specify the printer name to send it to a different printer
- Use the
GetPrintDocument
method to specify Microsoft PrinterSettings and PrintController
Install with NuGet
Install-Package IronPdf
Print PDF File Example
The Print
method can be accessed from the PdfDocument object. Both newly rendered and existing PDF files can be printed using this method. Invoke the Print
method to print a PDF using the default printer of the machine. However, you can print to a specific printer by providing the printer name as a string to the Print
method.
Please note
:path=/static-assets/pdf/content-code-examples/how-to/print-pdf-print.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>");
// Send the document to "Microsoft Print to PDF" printer
pdf.Print("Microsoft Print to PDF");
Imports IronPdf
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>")
' Send the document to "Microsoft Print to PDF" printer
pdf.Print("Microsoft Print to PDF")
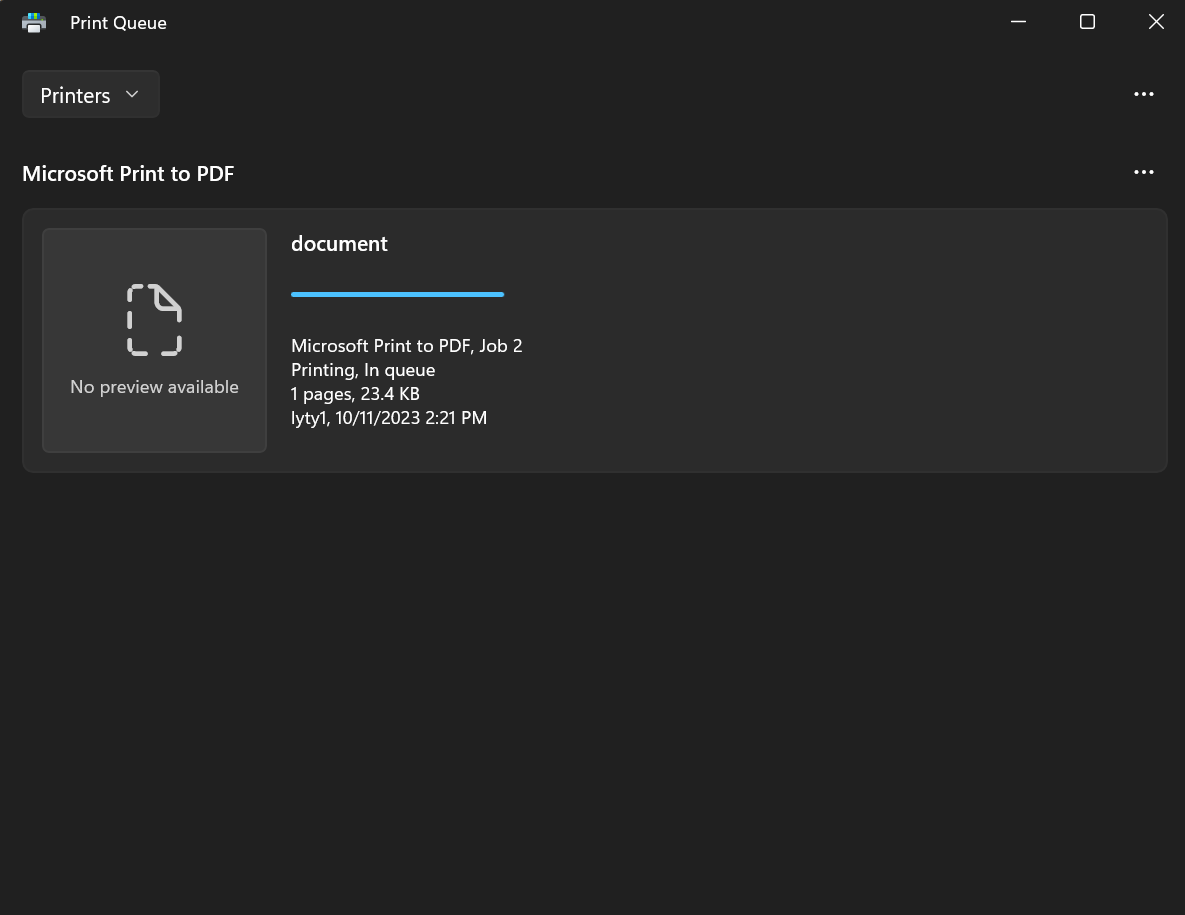
Printer Resolution
Specify the resolution of the printed PDF file by passing the desired DPI number into the Print
method. This will set an equal DPI for both vertical and horizontal. In the case that different DPI values are desired for the vertical and horizontal, you can specify two parameters, where the first number will be for the horizontal (x), and the second for the vertical (y).
:path=/static-assets/pdf/content-code-examples/how-to/print-pdf-dpi.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>");
// Set custom DPI
pdf.Print(300);
// Specify printing resolution
pdf.Print(10, 10, "Microsoft Print to PDF");
Imports IronPdf
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>")
' Set custom DPI
pdf.Print(300)
' Specify printing resolution
pdf.Print(10, 10, "Microsoft Print to PDF")
Let's see how to rasterize and print PDF file in the next example.
Print to File
The PrintToFile
method is a quick way to rasterize PDF documents, converting them into a bitmap (pixel-based) image format before saving them as a PDF file. This rasterization is performed by the Windows built-in printer; in my case, it's the "Microsoft Print to PDF." This method only prints the PDF file to the disk and does not send it to a physical printer.
:path=/static-assets/pdf/content-code-examples/how-to/print-pdf-print-to-file.cs
using IronPdf;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>");
// Print to file
pdf.PrintToFile("");
Imports IronPdf
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Test printing</h1>")
' Print to file
pdf.PrintToFile("")
Explore Print PDF Document Settings
To configure the printing options further, use the GetPrintDocument
method, which accepts both Microsoft PrinterSettings and PrintController. The GetPrintDocument
method returns the current print document object. PrinterSettings options are described below the code example, while PrintController can be used to customize how printing is executed and provides options for handling exceptions and progress reporting, such as the print dialog, print preview, tracing printing processes, and other printing-related tasks.
:path=/static-assets/pdf/content-code-examples/how-to/print-pdf-printer-setting.cs
using IronPdf;
using System.Drawing.Printing;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>Testing</h1>");
PrinterSettings settings = new PrinterSettings() {
PrinterName = "Microsoft Print to PDF",
// Number of Copy
Copies = 2,
// Page range to print
FromPage = 2,
ToPage = 4,
};
PrintDocument document = pdf.GetPrintDocument(settings);
// Print
document.Print();
Imports IronPdf
Imports System.Drawing.Printing
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>Testing</h1>")
Private settings As New PrinterSettings() With {
.PrinterName = "Microsoft Print to PDF",
.Copies = 2,
.FromPage = 2,
.ToPage = 4
}
Private document As PrintDocument = pdf.GetPrintDocument(settings)
' Print
document.Print()
- CanDuplex: Indicates whether the printer supports duplex (double-sided) printing. If true, printing on both sides of the paper is possible; otherwise, it cannot.
- Collate: Specifies whether multiple pdf files or copies of a pdf document should be collated (organized in order) when printed. When true, the printer collates the copies; when false, it does not.
- Copies: Sets the number of copies of the PDF document to print. It determines how many identical copies of the document will be printed.
- DefaultPageSettings: Represents the default page settings for the printer, including paper size, margins, and orientation.
- Duplex: Specifies the duplex (double-sided) printing mode to use. Options include Duplex.Default, Duplex.Simplex (single-sided), Duplex.Horizontal, and Duplex.Vertical.
- InstalledPrinters: Provides a collection of installed printer names on the system. You can iterate through this collection to get the names of available printers.
- IsDefaultPrinter: Indicates whether the printer specified in PrinterName is set as the default printer on the system.
- IsPlotter: Determines whether the printer is a plotter. Plotter printers are often used for large-format printing, such as for architectural or engineering drawings.
- IsValid: Indicates whether the printer settings are valid and can be used for printing PDF files.
- LandscapeAngle: Specifies the angle (rotation) of landscape orientation for the printer, usually 90 degrees for portrait.
- MaximumCopies: Represents the maximum number of copies that can be specified for printing PDF.
- MaximumPage: Specifies the maximum page number that can be set for printing or conversion.
- MinimumPage: Specifies the minimum page number that can be set for printing or conversion.
- PaperSizes: Provides a collection of supported paper sizes for the printer. You can query this collection to determine available paper sizes.
- PaperSources: Offers a collection of paper sources or trays available for the printer. This can be useful when selecting the paper source for printing PDF files.
- PrinterName: Specifies the name of the printer to use for printing or conversion.
- PrinterResolutions: Provides a collection of available printer resolutions, allowing you to choose the print quality.
- PrintFileName: Gets or sets the file name when printing to a file using PrintToFile.
- PrintRange: Specifies the range of PDF pages to print, such as all pages, a specific range, or a selection. Use this to print specific pages.
- FromPage: Specifies the starting page number for printing or conversion. Printing will begin from this page.
- ToPage: Specifies the ending page number for printing or conversion. Printing will stop after reaching this page.
- PrintToFile: Indicates whether to print to a file instead of a physical printer. When true, you can specify the file path using PrintFileName.
- SupportsColor: Indicates whether the printer supports color printing. If true, print in color is supported; otherwise, it is limited to black and white (monochrome) printing.
Lastly to configure the default printer to print PDF, you may go to the "Printers & Scanners" section of the machine setting.