Add or Avoid Page Breaks in HTML PDFs
IronPDF supports page breaks within PDF documents. One major difference between PDF documents and HTML is that HTML documents tend to scroll whereas PDFs are multi paged and can be printed.
How to Use Page Breaks in HTML PDFs
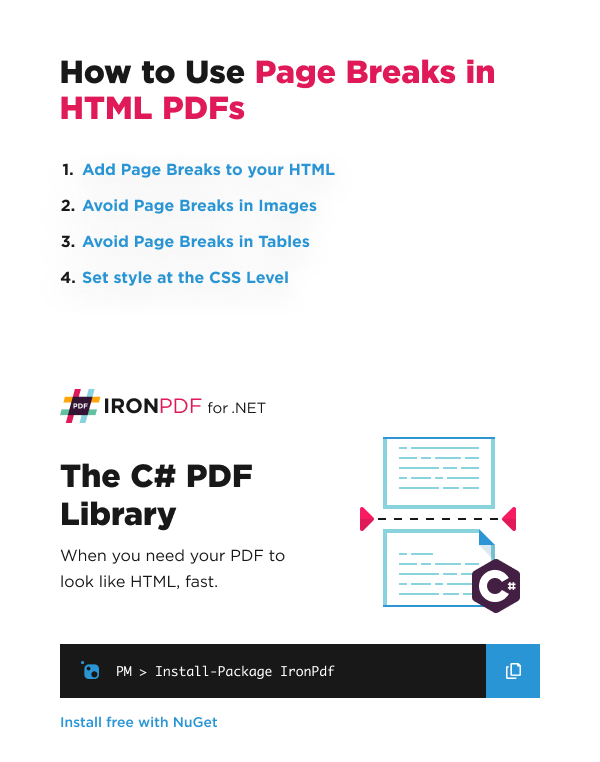
Install with NuGet
Install-Package IronPdf
Add a Page Break
To create a page-break in HTML you can use this in your HTML code:
<div style='page-break-after: always;'> </div>
Demonstration of Create a Page Break
In this example I have the following table and image in my HTML and I would like them to be on two seperate pages by adding a page break after the table.
Table
Company | Product |
---|---|
Iron Software | IronPDF |
Iron Software | IronOCR |
Image
:path=/static-assets/pdf/content-code-examples/how-to/html-to-pdf-page-breaks-page-break.cs
using IronPdf;
const string html = @"
<table style='border: 1px solid #000000'>
<tr>
<th>Company</th>
<th>Product</th>
</tr>
<tr>
<td>Iron Software</td>
<td>IronPDF</td>
</tr>
<tr>
<td>Iron Software</td>
<td>IronOCR</td>
</tr>
</table>
<div style='page-break-after: always;'> </div>
<img src='https://ironpdf.com/img/products/ironpdf-logo-text-dotnet.svg'>";
var renderer = new ChromePdfRenderer();
var pdf = renderer.RenderHtmlAsPdf(html);
pdf.SaveAs("Page_Break.pdf");
Imports IronPdf
Private Const html As String = "
<table style='border: 1px solid #000000'>
<tr>
<th>Company</th>
<th>Product</th>
</tr>
<tr>
<td>Iron Software</td>
<td>IronPDF</td>
</tr>
<tr>
<td>Iron Software</td>
<td>IronOCR</td>
</tr>
</table>
<div style='page-break-after: always;'> </div>
<img src='https://ironpdf.com/img/products/ironpdf-logo-text-dotnet.svg'>"
Private renderer = New ChromePdfRenderer()
Private pdf = renderer.RenderHtmlAsPdf(html)
pdf.SaveAs("Page_Break.pdf")
And the code above will generate the PDF below which has 2 pages, the Table on the First page and the Image on the second:
Avoiding page breaks in Images
To avoid a page-break within an image or table you may use the css page-break-inside attribute applied to a wrapping DIV element.
<div style='page-break-inside: avoid'>.
<img src='no-break-me.png'>
</div>
<div style='page-break-inside: avoid'>.
<img src='no-break-me.png'>
</div>
Avoiding page breaks in Tables
As shown above, page breaks within tables can be avoided by using the CSS:
page-break-inside: avoid
. This is better applied to a wrapping DIV than to the table itself to ensure the style is applied to a block level html node.
To duplicate table headers and footers across every page of a large HTML table spanning multiple pdf pages you may use an <thead>
group within the table:
<thead>
<tr>
<th>C Sharp</th><th>VB</th>
</tr>
</thead>
<thead>
<tr>
<th>C Sharp</th><th>VB</th>
</tr>
</thead>
Advanced CSS3 Settings
To give greater control you may wish to use CSS3 in addition to your thead
group:
<style type="text/css">
table { page-break-inside:auto }
tr { page-break-inside:avoid; page-break-after:auto }
thead { display:table-header-group }
tfoot { display:table-footer-group }
</style>
<style type="text/css">
table { page-break-inside:auto }
tr { page-break-inside:avoid; page-break-after:auto }
thead { display:table-header-group }
tfoot { display:table-footer-group }
</style>