How to Export PDF/A Format Documents in C#
IronPDF supports export of PDFs to the PDF/A-3b standard. PDF/A-3B is a strict subset of the ISO PDF specification used to create archival versions of documents with the intent that they will always render exactly the same as when they were saved.
Section 508 Compliance
IronPDF are happy to follow Googles Initiative to increase PDF Archiving and Accessibility and the Section 508 Compliance of PDF documents.
In 2021 we moved to rendering PDFs from HTML using the Google Chromium HTML rendering engine. This allows our software to inherit the accessibility work Google have already implemented: https://blog.chromium.org/2020/07/using-chrome-to-generate-more.html
How to Convert PDF to PDFA in C#
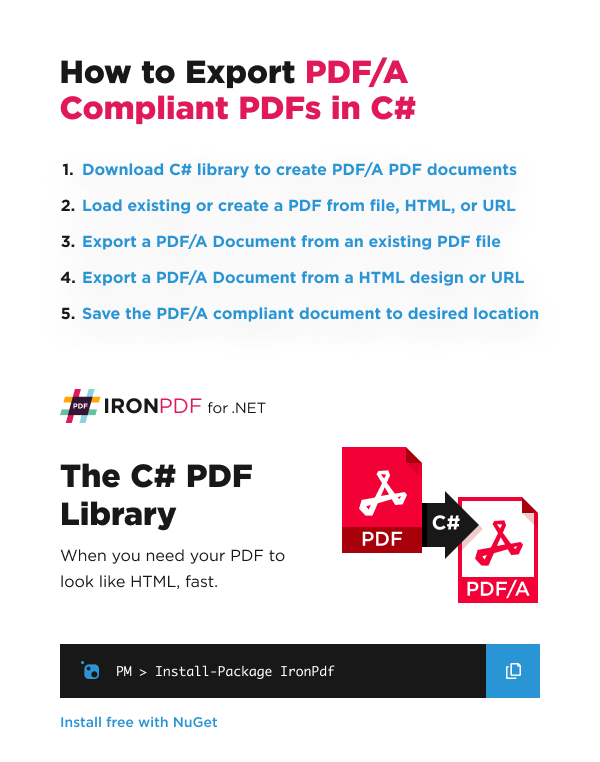
- Download C# library to create PDF/A PDF documents
- Load existing or create a PDF from file, HTML, or URL
- Export a PDF/A Document from an existing PDF file
- Export a PDF/A Document from a HTML design or URL
- Save the PDF/A compliant document to desired location
Install with NuGet
Install-Package IronPdf
From an Existing PDF File
I have an example PDF "wikipedia.pdf
" which was generated using IronPDF and saved as a PDF file.
I will load and re-save it as a PDF/A-3B compliant PDF file in this demonstration.
Input file: "wikipedia.pdf"
Code
:path=/static-assets/pdf/content-code-examples/how-to/pdfa-fromfile.cs
using IronPdf;
// Create a PdfDocument object or open any PDF File
PdfDocument pdf = PdfDocument.FromFile("wikipedia.pdf");
// Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("pdf-a3-wikipedia.pdf", PdfAVersions.PdfA3);
Imports IronPdf
' Create a PdfDocument object or open any PDF File
Private pdf As PdfDocument = PdfDocument.FromFile("wikipedia.pdf")
' Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("pdf-a3-wikipedia.pdf", PdfAVersions.PdfA3)
Output
The output file is PDF/A-3b compliant:
From a HTML Design or URL
I have an example HTML design "design.html
" which I would like to render from HTML to PDF using IronPDF and then export as a PDF/A compliant file.
I will save it as a PDF/A-3B compliant PDF file in this demonstration.
HTML Design Example
:path=/static-assets/pdf/content-code-examples/how-to/pdfa-fromhtml.cs
using IronPdf;
// Use the Chrome Renderer to make beautiful HTML designs
var chromeRenderer = new ChromePdfRenderer();
// Render an HTML design as a PdfDocument object using Chrome
PdfDocument pdf = chromeRenderer.RenderHtmlAsPdf("design.html");
// Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("design-accessible.pdf", PdfAVersions.PdfA3);
Imports IronPdf
' Use the Chrome Renderer to make beautiful HTML designs
Private chromeRenderer = New ChromePdfRenderer()
' Render an HTML design as a PdfDocument object using Chrome
Private pdf As PdfDocument = chromeRenderer.RenderHtmlAsPdf("design.html")
' Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("design-accessible.pdf", PdfAVersions.PdfA3)
The output file is PDF/A-3B compliant:
URL Example
I have the following website "https://www.microsoft.com
" which I would like to render from URL to PDF using IronPDF and then export as a PDF/A compliant file.
I will save it as a PDF/A-3B compliant PDF file in this demonstration.
:path=/static-assets/pdf/content-code-examples/how-to/pdfa-fromurl.cs
using IronPdf;
// Use the Chrome Renderer to make beautiful HTML designs from URLs
var chromeRenderer = new ChromePdfRenderer();
// Render a Website as a PdfDocument object using Chrome
PdfDocument pdf = chromeRenderer.RenderUrlAsPdf("https://www.microsoft.com");
// Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("website-accessible.pdf", PdfAVersions.PdfA3);
Imports IronPdf
' Use the Chrome Renderer to make beautiful HTML designs from URLs
Private chromeRenderer = New ChromePdfRenderer()
' Render a Website as a PdfDocument object using Chrome
Private pdf As PdfDocument = chromeRenderer.RenderUrlAsPdf("https://www.microsoft.com")
' Use the SaveAsPdfA method to save to file
pdf.SaveAsPdfA("website-accessible.pdf", PdfAVersions.PdfA3)
The output file is PDF/A-3B compliant: