Digitally Sign a PDF Document
Important Disambiguation: Signing a PDF
Developers commonly ask how they can programmatically add a signature to a PDF document using IronPDF. Commonly, signing means different things to different developers:
- To digitally sign a PDF document with a Certificate to ensure it can not been tampered with.
- To add a graphical handwritten signature image to an existing PDF from an image file.
- To stamp a Certificate's Image onto a PDF.
- To add a Signature Form Field to a PDF which some viewers can prompt for Signing.
Install with NuGet
Install-Package IronPdf
How to Sign PDF File in C#
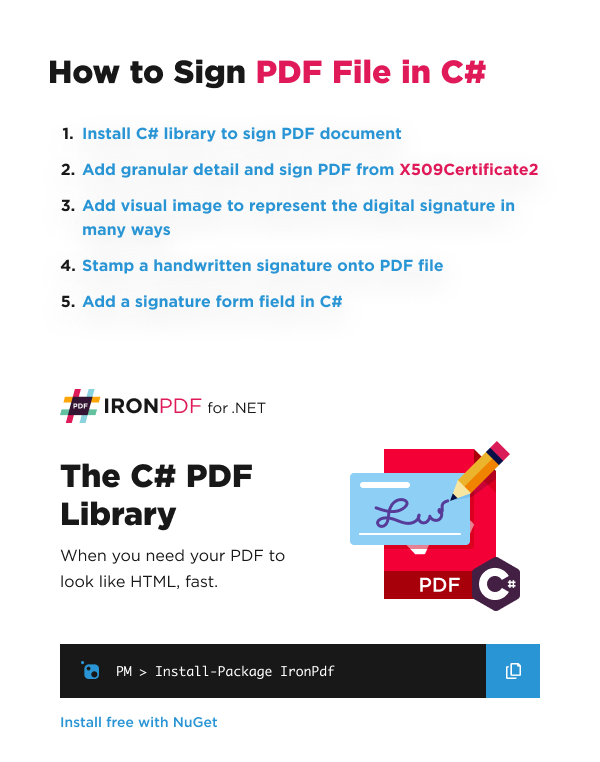
Sign a PDF with a Digital Certificate
IronPDF supports many ways to sign a PDF with a digital signature certificate of .pfx
and .p12
formats. In this How-To guide, you will be guided through all three main methods used to digitally sign PDF document:
Signing Method | Description |
---|---|
Sign | Sign a PDF with a PdfSignature object |
SignWithFile | Sign PDF with a digital signature certificate(.pfx or .p12) on disk |
SignWithStore | Signs the PDF with digital signature extracted from your computer's signature storage. Based on a thumbprint ID |
Supported Digital Signature Certificate Files
We officially comply to the X509Certificate2
standard and support .pfx
and .p12
signatures. If your signature is unable to be applied directly in IronPDF's signing methods, you will need to create a X509Certificate2
certificate with instructions that can be found here.
Sign: Create PdfSignature From X509Certificate2
IronPDF's Signing methods will accept X509Certificate2
variable with X509KeyStorageFlags set to Exportable.
Please Note
- IronPDF only support X509KeyStorageFlags.Exportable. Some certificate have KeyStorageFlags set to Exportable by defualt.
- Attempting to use different KeyStorageFlags will result in exception => Internal.Cryptography.CryptoThrowHelper.WindowsCryptographicException: 'The requested operation is not supported.'
:path=/static-assets/pdf/content-code-examples/how-to/signing-X509Certificate2-with-privatekey.cs
using IronPdf;
using IronPdf.Signing;
using System.Security.Cryptography.X509Certificates;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>foo</h1>");
// Create X509Certificate2 object with X509KeyStorageFlags set to Exportable
X509Certificate2 cert = new X509Certificate2("IronSoftware.pfx", "123456", X509KeyStorageFlags.Exportable);
// Create PdfSignature object
var sig = new PdfSignature(cert);
// Sign PDF document
pdf.Sign(sig);
pdf.SaveAs("signed.pdf");
Imports IronPdf
Imports IronPdf.Signing
Imports System.Security.Cryptography.X509Certificates
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>foo</h1>")
' Create X509Certificate2 object with X509KeyStorageFlags set to Exportable
Private cert As New X509Certificate2("IronSoftware.pfx", "123456", X509KeyStorageFlags.Exportable)
' Create PdfSignature object
Private sig = New PdfSignature(cert)
' Sign PDF document
pdf.Sign(sig)
pdf.SaveAs("signed.pdf")
Sign: Add Granular Detail to PdfSignature
While instantiating PdfSignature or after instantiated, useful information can be added to the PdfSignature object including date, signing contact, location, signing reason, timestampe, and adding image as a visual appearance on the PDF document.
Tips
:path=/static-assets/pdf/content-code-examples/how-to/signing-add-granular-information.cs
using IronPdf;
using IronPdf.Signing;
using IronSoftware.Drawing;
using System;
ChromePdfRenderer renderer = new ChromePdfRenderer();
PdfDocument pdf = renderer.RenderHtmlAsPdf("<h1>foo</h1>");
pdf.SaveAs("signed.pdf");
// Create PdfSignature object
var sig = new PdfSignature("IronSoftware.pfx", "123456");
// Add granular information
sig.SignatureDate = new DateTime(2000, 12, 02);
sig.SigningContact = "IronSoftware";
sig.SigningLocation = "Chicago";
sig.SigningReason = "How to guide";
sig.TimestampHashAlgorithm = TimestampHashAlgorithms.SHA256;
sig.TimeStampUrl = "http://timestamp.digicert.com";
sig.SignatureImage = new PdfSignatureImage("IronSoftware.png", 0, new Rectangle(0, 600, 100, 100));
// Sign and save PDF document
sig.SignPdfFile("signed.pdf");
Imports IronPdf
Imports IronPdf.Signing
Imports IronSoftware.Drawing
Imports System
Private renderer As New ChromePdfRenderer()
Private pdf As PdfDocument = renderer.RenderHtmlAsPdf("<h1>foo</h1>")
pdf.SaveAs("signed.pdf")
' Create PdfSignature object
Dim sig = New PdfSignature("IronSoftware.pfx", "123456")
' Add granular information
sig.SignatureDate = New DateTime(2000, 12, 02)
sig.SigningContact = "IronSoftware"
sig.SigningLocation = "Chicago"
sig.SigningReason = "How to guide"
sig.TimestampHashAlgorithm = TimestampHashAlgorithms.SHA256
sig.TimeStampUrl = "http://timestamp.digicert.com"
sig.SignatureImage = New PdfSignatureImage("IronSoftware.png", 0, New Rectangle(0, 600, 100, 100))
' Sign and save PDF document
sig.SignPdfFile("signed.pdf")
Demonstration
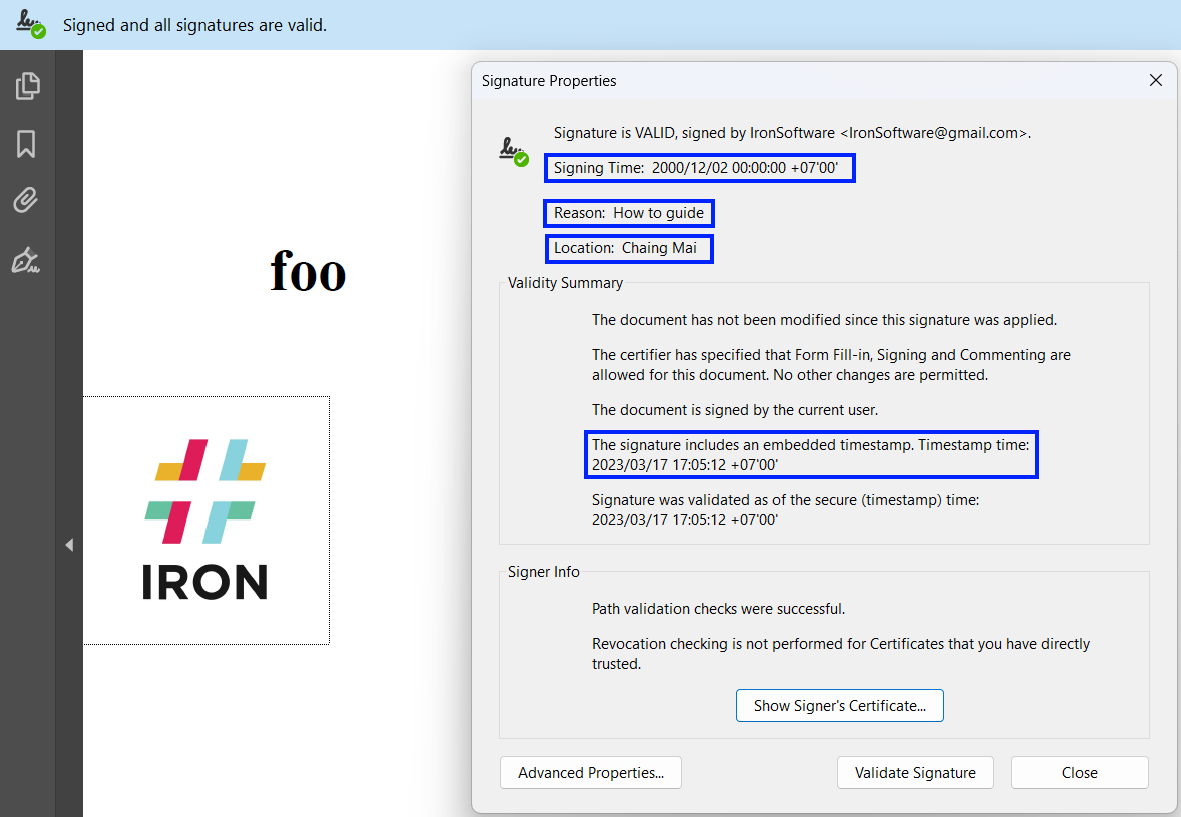
Different Ways to Add Image
Set SignatureImage property to a new PdfSignatureImage object.
:path=/static-assets/pdf/content-code-examples/how-to/signing-add-image-by-property.cs
using IronPdf.Signing;
using IronSoftware.Drawing;
// Create PdfSignature object
var sig = new PdfSignature("IronSoftware.pfx", "123456");
sig.SignatureImage = new PdfSignatureImage("IronSoftware.png", 0, new Rectangle(0, 600, 100, 100));
Imports IronPdf.Signing
Imports IronSoftware.Drawing
' Create PdfSignature object
Private sig = New PdfSignature("IronSoftware.pfx", "123456")
sig.SignatureImage = New PdfSignatureImage("IronSoftware.png", 0, New Rectangle(0, 600, 100, 100))
Use LoadSignatureImageFromFile
method to load image from file. The LoadSignatureImageFromFile support various image formats.
:path=/static-assets/pdf/content-code-examples/how-to/signing-add-image-from-file.cs
using IronPdf.Signing;
using IronSoftware.Drawing;
// Create PdfSignature object
var sig = new PdfSignature("IronSoftware.pfx", "123456");
sig.LoadSignatureImageFromFile("IronSoftware.png", 0, new Rectangle(0, 600, 100, 100));
Imports IronPdf.Signing
Imports IronSoftware.Drawing
' Create PdfSignature object
Private sig = New PdfSignature("IronSoftware.pfx", "123456")
sig.LoadSignatureImageFromFile("IronSoftware.png", 0, New Rectangle(0, 600, 100, 100))
Use LoadSignatureImageFromStream
method to load image from stream. The image stream can be generated from other library as long as it has the format of TGA, PBM, TIFF , BMP, GIF, PNG,JPEG, Webp.
:path=/static-assets/pdf/content-code-examples/how-to/signing-add-image-from-stream.cs
using IronPdf.Signing;
using IronSoftware.Drawing;
// Create PdfSignature object
var sig = new PdfSignature("IronSoftware.pfx", "123456");
// Import image using IronSoftware.Drawing
AnyBitmap image = AnyBitmap.FromFile("IronSoftware.png");
sig.LoadSignatureImageFromStream(image.ToStream(), 0, new Rectangle(0, 600, 100, 100));
Imports IronPdf.Signing
Imports IronSoftware.Drawing
' Create PdfSignature object
Private sig = New PdfSignature("IronSoftware.pfx", "123456")
' Import image using IronSoftware.Drawing
Private image As AnyBitmap = AnyBitmap.FromFile("IronSoftware.png")
sig.LoadSignatureImageFromStream(image.ToStream(), 0, New Rectangle(0, 600, 100, 100))
Signature Permissions
If you choose, you may explicitly specify conditions that your Certificate will remain valid. If you want your Signature invalidated upon and change, or allowing just form field changes, etc. Please use the following table to see the options:
PdfDocument.SignaturePermissions | Definition |
---|---|
NoChangesAllowed | No changes are allowed |
FormFillingAllowed | Changing form field values are allowed |
FormFillingAndAnnotationsAllowed | Changing form field values and modifying annotations are allowed |
This parameter is optional and not setting it will apply a signature that certifies a specific revision and cannot be invalidated.
Save and Sign a PDF Revision Iteration
In the following example we open a PDF file, make various edits, then before we save we will sign it. For signature permissions, we will only allow form-filling as future edits, otherwise the signature will be invalidated from any other edit.
We will then call SaveAsRevision
to save the revision to the history and then save our new document to disk.
:path=/static-assets/pdf/content-code-examples/how-to/signing-revision.cs
using IronPdf;
using IronPdf.Rendering;
// Import PDF and enable TrackChanges
PdfDocument pdf = PdfDocument.FromFile("annual_census.pdf", TrackChanges: ChangeTrackingModes.EnableChangeTracking);
// ... various edits ...
pdf.SignWithFile("/assets/IronSignature.p12", "password", null, IronPdf.Signing.SignaturePermissions.FormFillingAllowed);
PdfDocument pdfWithRevision = pdf.SaveAsRevision();
pdfWithRevision.SaveAs("annual_census_2.pdf");
Imports IronPdf
Imports IronPdf.Rendering
' Import PDF and enable TrackChanges
Private pdf As PdfDocument = PdfDocument.FromFile("annual_census.pdf", TrackChanges:= ChangeTrackingModes.EnableChangeTracking)
' ... various edits ...
pdf.SignWithFile("/assets/IronSignature.p12", "password", Nothing, IronPdf.Signing.SignaturePermissions.FormFillingAllowed)
Dim pdfWithRevision As PdfDocument = pdf.SaveAsRevision()
pdfWithRevision.SaveAs("annual_census_2.pdf")
Understanding Incremental Saving for Signatures
While some viewers like Chrome browser only show one version, PDF files have the capability to store previous versions of the document similar to a Git commit history. You will see this in more advanced PDF viewers such as Adobe Acrobat.
When dealing with PDF signatures, it is important to know about this because the action of signing a PDF applies to the current iteration of the PDF. Your PDF may have signatures for older iterations, or may have a few unsigned versions. We can visualize an example like follows:
PDF Document Iteration | Certificate A | Certificate B | Certificate C | Certificate D |
---|---|---|---|---|
0 (first save) | ✅ | |||
1 | ||||
2 | ||||
3 | ✅ (form field edits only) | ✅ (form field edits only) | ||
4 (only form fields edited) | ✅ | |||
5 | ✅ (no further edits allowed) | ✅ (no further edits allowed) | ✅ (no further edits allowed) |
Above, we have a documents that has been through 6 different iterations. This document may be being passed around departments of a company with approval until being finalized at iteration 3. In this iteration, both Person A and Person B signed the document with the permission "Form Field Edits Only" set. This means that filling in form fields in the PDF document is allowed, but any other change to the document will invalidate their signatures.
In the example above we can assume Person C is the one who has filled out the form and sent it back to Person A, C, and D who all signed the document a final time with the "No Edits Allowed" permission. Because no illegal actions were taken in this document invalidating signatures, when we run IronPDF's signature method we will get true
.
Roll back to an Old Revision
To roll back to a previous revision of a PDF, you can use the GetRevision
method. This will forget any changes made since this revision including newer signatures. To do this use:
:path=/static-assets/pdf/content-code-examples/how-to/signing-revert-revision.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("report.pdf");
int versions = pdf.RevisionCount; // total revisions
PdfDocument rolledBackPdf = pdf.GetRevision(2);
rolledBackPdf.SaveAs("report-draft.pdf");
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("report.pdf")
Private versions As Integer = pdf.RevisionCount ' total revisions
Private rolledBackPdf As PdfDocument = pdf.GetRevision(2)
rolledBackPdf.SaveAs("report-draft.pdf")
Remove Signatures
IronPDF has a method RemoveSignatures
that will remove every signature of every revision for a PDF document. Usage is as follows:
:path=/static-assets/pdf/content-code-examples/how-to/signing-remove-signature.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("invoice.pdf");
pdf.RemoveSignatures();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("invoice.pdf")
pdf.RemoveSignatures()
Verify All Signatures in a PDF
Calling the verify signatures method on a PDF document will look at all Signatures from all document iterations and verify that all of them are still valid. This will return a bool
of true
if they are all valid.
:path=/static-assets/pdf/content-code-examples/how-to/signing-verify-signatures.cs
using IronPdf;
PdfDocument pdf = PdfDocument.FromFile("annual_census.pdf");
bool isValid = pdf.VerifyPdfSignatures();
Imports IronPdf
Private pdf As PdfDocument = PdfDocument.FromFile("annual_census.pdf")
Private isValid As Boolean = pdf.VerifyPdfSignatures()
Stamp a Signature onto a PDF
First off, I will start with a PDF I want to sign. I will use this example invoice:
We will apply a handwritten signature which is in the form of a .png
image to our PDF. This may be a handwritten signature or the image that was used in the creation of a certificate file. This is the sample signature we will use:
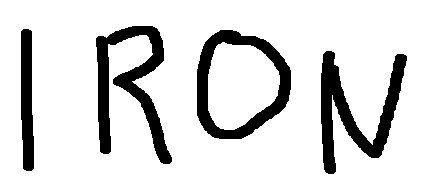
Code
With this we will use the following code to stamp the handwritten signature to the PDF as a watermark:
:path=/static-assets/pdf/content-code-examples/how-to/signing-handwritten.cs
using IronPdf;
using IronPdf.Editing;
var pdf = PdfDocument.FromFile("invoice.pdf");
pdf.ApplyWatermark("<img src='signature.png'/>", 90, VerticalAlignment.Bottom, HorizontalAlignment.Right);
pdf.SaveAs("official_invoice.pdf");
Imports IronPdf
Imports IronPdf.Editing
Private pdf = PdfDocument.FromFile("invoice.pdf")
pdf.ApplyWatermark("<img src='signature.png'/>", 90, VerticalAlignment.Bottom, HorizontalAlignment.Right)
pdf.SaveAs("official_invoice.pdf")
Output Result
After this code is run, we have this output file which has our signature on the bottom right:
Add an Unsigned Signature Field to a PDF
This is not currently supported yet. You can read more about IronPDF and the forms it supports in the Programmatically Fill PDF Forms in C# article.