ASPX Pages to PDF in ASP.NET
This ASPX to PDF tutorial will guide you step-by-step on how to convert ASPX to PDF: save an ASPX page as a PDF in ASP.NET web applications.
Users should never have to open the ASPX file with the .aspx file extension in Google Chrome. We ask our engineering team to convert ASPX to PDF automatically using .NET code! We never need to press CTRL P! There is a server-based way to convert ASPX internet media and save as PDF.
Apply settings including setting file behavior and names, adding headers & footers, changing print options, adding page breaks, combining Async & Multithreading and more.
Converting ASPX files to PDF in simple steps
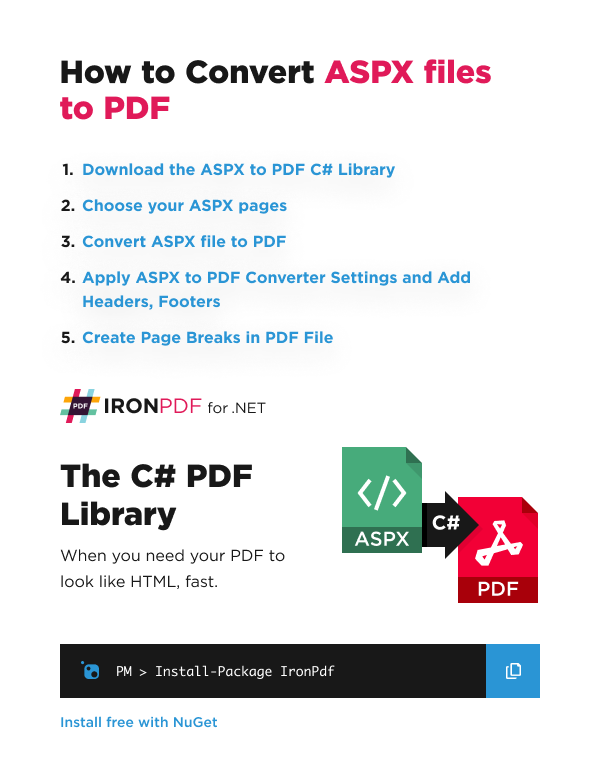
- Download the ASPX to PDF C# Library
- Choose your ASPX pages
- Convert ASPX file to PDF
- Apply ASPX to PDF Converter Settings and Add Headers, Footers
- Create Page Breaks in PDF File
How to convert ASPX files to PDF
Microsoft Web Form Applications for ASP.NET are commonly used in the development of sophisticated websites, online banking, intranets and accounting systems. One common feature of ASP.NET (ASPX) websites is to generate dynamic PDF files such as invoices, tickets, or management reports for users to download in PDF format.
This tutorial shows how to use the IronPDF software component for .NET to turn any ASP.NET web form into a PDF (ASP.NET to PDF). HTML, normally rendered as a web page, will be used to render as a PDF for download or viewing in a web browser. The attached source project will show you how to convert a webpage to PDF in ASP.NET using C#.
We achieve this HTML to PDF conversion (convert ASPX to PDF) when rendering webpages using the IronPDF and its AspxToPdf tools Class.
1. Install the ASPX file Converter Free from IronPDF
Install with NuGet
Install-Package IronPdf
Install via NuGet
In Visual Studio, right click on your project solution explorer and select "Manage NuGet Packages...". From there simply search for IronPDF and install the latest version... click ok to any dialog boxes that come up.
This will work in any C# .NET Framework project from Framework 4 and above, or .NET Core 2 and above. It will also work just as well in VB.NET projects.
Install-Package IronPdf
https://www.nuget.org/packages/IronPdf
Install via DLL
Alternatively, the IronPDF DLL can be downloaded and manually installed to the project or GAC from https://ironpdf.com/packages/IronPdf.zip
Remember to add this statement to the top of any cs class file using IronPDF:
using IronPdf;
2. Convert ASP.NET Webpages to PDF
We start with a normal ASPX "Web Form," which renders as HTML. We later convert the ASPX page to PDF file format.
In the attached example source code, we rendered a business invoice "Invoice.aspx," a simple HTML business invoice rendered as an ASP.NET Page.
The HTML page contains CSS3 stylesheets and may also include images and javascript.
To render this ASP.NET Web Page to a PDF instead of HTML, we need to open the C# (or VB.NET) code and add this to the Page_Load event:
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-1.cs
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.InBrowser);
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.InBrowser)
This is all that's required; the HTML now renders as a PDF. Hyperlinks, StyleSheets, Images and even HTML forms are preserved. This is very similar to the output if the user printed the HTML to a PDF in their browser themselves. IronPDF is built upon Chromium web browser technology that powers Google Chrome.
The entire C# code reads like this in full: Convert The ASPX Page as PDF in Active Server Pages.
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-2.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using IronPdf;
namespace AspxToPdfTutorial
{
public partial class Invoice : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.InBrowser);
}
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web
Imports System.Web.UI
Imports System.Web.UI.WebControls
Imports IronPdf
Namespace AspxToPdfTutorial
Partial Public Class Invoice
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.InBrowser)
End Sub
End Class
End Namespace
3. Apply ASPX File to PDF Converter Settings
There are many options to tweak and perfect when we convert an ASPX file to PDF generated using .NET Web Forms.
These options are documented in full online at https://ironpdf.com/object-reference/api/IronPdf.AspxToPdf.html
3.1. Set PDF File Behavior
"InBrowser" file behavior attempts to show the PDF directly in the user's browser. This is not always possible in every web browser, but typically a common feature of modern, standards-compliant browsers.
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.InBrowser);
"Attachment" file behavior causes the PDF to be downloaded.
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment);
3.2. Set PDF File Name
We may also set the file name of the PDF document by adding an additional parameter. This means we can control the name of the file when the user decides to download or keep it. When we save the ASPX page as a PDF, this name will be given to the PDF document.
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "Invoice.pdf");
3.3. Change PDF Print Options
We can control the output of the PDF by adding an instance of the IronPdf.ChromePdfRenderer Class:
https://ironpdf.com/object-reference/api/IronPdf.ChromePdfRenderer.html
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-3.cs
var AspxToPdfOptions = new IronPdf.ChromePdfRenderOptions()
{
EnableJavaScript = false,
//.. many more options available
};
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "Invoice.pdf", AspxToPdfOptions);
Dim AspxToPdfOptions = New IronPdf.ChromePdfRenderOptions() With {.EnableJavaScript = False}
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "Invoice.pdf", AspxToPdfOptions)
The PDF rendering options available include:
- ApplyMarginToHeaderAndFooter. Apply margin option to HTML Headers and Footers. default is false which make HTML Headers and Footers have 0 margin. Only support ChromeRender.
- CreatePdfFormsFromHtml. Turns ASPX form elements into editable PDF forms.
- CssMediaType. Enables Media="screen" or "print" for CSS Styles and CSS3 StyleSheets.
- CustomCssUrl. Allows a custom CSS style-sheet to be applied to HTML by URL.
- EnableJavaScript. Enables JavaScript, jQuery and even Json code within the ASPX Page. A RenderDelay may need to be applied.
- FirstPageNumber. First page number for Header and Footer. The default is 1.
- FitToPaperWidth. Where possible, shrinks the PDF content to a width of 1 page of virtual paper.
- GenerateUniqueDocumentIdentifiers. Set to false if you wish to use binary file equality to compare PDFs such as for Unit Tests.
- GrayScale. Outputs a greyscale PDF in shades of grey instead of full color.
- HtmlHeader. Sets the header content for every PDF page using content strings or even HTML.
- TextHeader. Sets the footer content for every PDF page as text. Supports 'mail-merge' and automatically turns urls into hyperlinks.
- HtmlFooter. Sets the footer content for every PDF page using content strings or even HTML.
- TextFooter. Sets the header content for every PDF page as text. Supports 'mail-merge' and automatically turns urls into hyperlinks.
- InputEncoding. The input character encoding as a string. UTF-8 is Default for ASP.NET.
- MarginBottom. Bottom PDF Paper margin in millimeters. Set to zero for a borderless pdf.
- MarginLeft. Left PDF Paper margin in millimeters. Set to zero for a borderless pdf.
- MarginRight. Right PDF Paper margin in millimeters. Set to zero for a borderless pdf.
- MarginTop. Top PDF Paper margin in millimeters. Set to zero for a borderless pdf.
- PaperOrientation. The PDF paper orientation. Landscape or Portrait.
- PaperSize. Set an output paper size for PDF pages using System.Drawing.Printing.PaperKind. Alternatively developers may use the SetCustomPaperSize(int width, int height) method to create custom sizes.
- PrintHtmlBackgrounds. Prints HTML image backgrounds.
- RenderDelay. Milliseconds to wait after HTML is rendered before printing so that Javacsript or JSON have time to work.
- Timeout. Render timeout in seconds.
- Title. PDF Document 'Title' meta-data.
- ViewPortHeight. Defines a virtual screen height used to render HTML to PDF in IronPdf. Measured in pixels.
- ViewPortWidth. Defines a virtual screen width used to render HTML to PDF in IronPdf. Measured in pixels.
- Zoom. A % Scale level allowing the developer to enlarge or shrink html content.
4. Add Headers & Footers to ASPX PDFs
Using IronPDF, Headers and Footers can be added to the PDF output.
The simplest way to do this is with the SimpleHeaderFooter class, which supports a basic layout that can easily add dynamic data such as the current time and page numbering.
4.1. ASPX to PDF Header & Footer Example
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-4.cs
using IronSoftware.Drawing;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace AspxToPdfTutorial
{
public partial class Invoice : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
var AspxToPdfOptions = new IronPdf.ChromePdfRenderOptions()
{
TextHeader = new IronPdf.TextHeaderFooter()
{
CenterText = "Invoice",
DrawDividerLine = false,
Font = FontTypes.Arial,
FontSize = 12
},
TextFooter = new IronPdf.TextHeaderFooter()
{
LeftText = "{date} - {time}",
RightText = "Page {page} of {total-pages}",
Font = IronSoftware.Drawing.FontTypes.Arial,
FontSize = 12,
},
};
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "Invoice.pdf", AspxToPdfOptions);
}
}
}
Imports IronSoftware.Drawing
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web
Imports System.Web.UI
Imports System.Web.UI.WebControls
Namespace AspxToPdfTutorial
Partial Public Class Invoice
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim AspxToPdfOptions = New IronPdf.ChromePdfRenderOptions() With {
.TextHeader = New IronPdf.TextHeaderFooter() With {
.CenterText = "Invoice",
.DrawDividerLine = False,
.Font = FontTypes.Arial,
.FontSize = 12
},
.TextFooter = New IronPdf.TextHeaderFooter() With {
.LeftText = "{date} - {time}",
.RightText = "Page {page} of {total-pages}",
.Font = IronSoftware.Drawing.FontTypes.Arial,
.FontSize = 12
}
}
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "Invoice.pdf", AspxToPdfOptions)
End Sub
End Class
End Namespace
Alternatively we can generate HTML headers and footers using the HtmlHeaderFooter class, which also supports CSS, images, and hyperlinks.
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-5.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace AspxToPdfTutorial
{
public partial class Invoice : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
var AspxToPdfOptions = new IronPdf.ChromePdfRenderOptions()
{
MarginTop = 50, // make sufficiant space for an HTML header
HtmlHeader = new IronPdf.HtmlHeaderFooter()
{
HtmlFragment = "<div style='text-align:right'><em style='color:pink'>page {page} of {total-pages}</em></div>"
}
};
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "MyDocument.pdf", AspxToPdfOptions);
}
}
}
Imports System
Imports System.Collections.Generic
Imports System.Linq
Imports System.Web
Imports System.Web.UI
Imports System.Web.UI.WebControls
Namespace AspxToPdfTutorial
Partial Public Class Invoice
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim AspxToPdfOptions = New IronPdf.ChromePdfRenderOptions() With {
.MarginTop = 50,
.HtmlHeader = New IronPdf.HtmlHeaderFooter() With {.HtmlFragment = "<div style='text-align:right'><em style='color:pink'>page {page} of {total-pages}</em></div>"}
}
IronPdf.AspxToPdf.RenderThisPageAsPdf(IronPdf.AspxToPdf.FileBehavior.Attachment, "MyDocument.pdf", AspxToPdfOptions)
End Sub
End Class
End Namespace
As seen in our examples, we may "merge" dynamic text or html into Headers / Footers using placeholders:
- {page} for the current page number of the PDF
- {total-pages} as the total number of pages within the PDF
- {date} for today's date in a format appropriate to the server's system environment
- {time} for the time in hours:seconds using a 24 hour clock
- {html-title} inserts the title from the head tag of the ASPX web form
- {pdf-title} for the document file name
5. Apply ASPX File to PDF Tricks: Page Breaks
Where as HTML commonly 'flows' into a long page, PDFs simulate digital paper and are broken into consistent pages. Adding the following code to your ASPX page will automatically create a page-break in the .NET generated PDF.
:path=/static-assets/pdf/content-code-examples/how-to/aspx-to-pdf-6.cs
<div style='page-break-after: always;'> </div>
6. Combine Async & Multithreading for Performance
IronPDF was built for .NET Framework 4.0, or .NET Core 2 or above. In Framework 4.5 or above projects, ASYNC can be utilized to improve performance when working with multiple documents.
Combining Async with multithreaded CPUs and the Paralllel.ForEach command will improve bulk PDF format processing significantly.
7. Download as ASP.NET Source Code
The full ASPX File to PDF Converter Source Code for this tutorial is available to be downloaded as a zipped Visual Studio Web Application project.
Download this tutorial as a ASP.NET Visual Studio project
The free download contains working code examples for a C# ASP.NET Web Forms project showing a web page rendered as a PDF with settings applied. We hope this tutorial has helped you to learn how to save an ASPX file as PDF.
Going Forwards
Generally, the best way to learn any programming technique is through experimentation within your own ASP.NET projects. This includes trying the ASPX to PDF Converter from IronPDF.
Developers may also be interested in the IronPdf.AspxToPdf Class reference: https://ironpdf.com/object-reference/api/IronPdf.AspxToPdf.html
8. Watch ASPX to PDF Tutorial Video
Tutorial Quick Access
Download this Tutorial as Source Code
The full ASPX File to PDF Converter Source Code for this tutorial is available as a zipped Visual Studio Web Application project. The free download contains working code examples for a C# ASP.NET Web Forms project, showing a web page rendered as a PDF with settings applied.
DownloadExplore this Tutorial on GitHub
The code for this C# ASPX-To-PDF project is available in C# and VB.NET on GitHub as a ASP.NET website project. Please go ahead and fork us on Github for more help using IronPDF. Feel free to share this with anyone who might be asking, 'How do I Convert ASPX to PDF?'
C# ASPX to PDF Website Project Advanced ASP.NET Page to PDF Samples in C# for creating PDFs ASP.NET PDF Examples in VB.NET for creating PDFsDownload C# PDF Quickstart guide
To make developing PDFs in your .NET applications easier, we have compiled a quick-start guide as a PDF document. This "Cheat-Sheet" provide quick access to common functions and examples for generating and editing PDFs in C# and VB.NET, and will help save time getting started using IronPDF in your .NET project.
DownloadView the API Reference
Explore the API Reference for IronPDF, outlining the details of all of IronPDF’s features, namespaces, classes, methods fields and enums.
View the API Reference